Hello. Today we will talk about using PWM with raspberry pi pico. With the help of a potentiometer, we will change the brightness of a led depending on the analog value we read.
What is PWM?
PWM stands for Pulse Width Modulation and it is a method that allows us to adjust the average power of the connected load and the device. In power electronics, they are often used in the control of mosfet and similar switching elements. The longer the switch stays open, the higher the total power transferred to the load will be.
The PWM signal depends on the ratio of on and off times of a square wave, and this ratio is called DUTY. While the duty ratio of the PWM signal changes, its Period does not change, that is, its frequency remains constant.
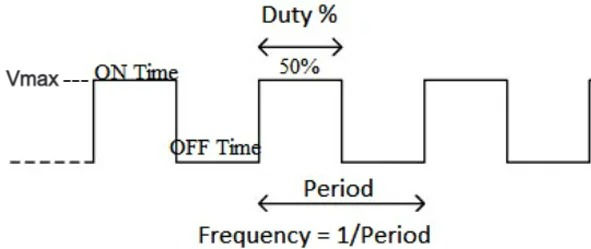
TON (open time): It is when the signal is high. For the Pico, this is 3.3 volts.
TOFF (off time): This is when the signal is low.
Period: It is the sum of open and closed times.
Duty Cycle: The percentage of time during a period that the signal is high. It is obtained by dividing the open time by the total time.
Duty=(Ton/(Ton+Toff)) * 100 // expanding by 100 for percent display
Vout = Vmax * Duty
Necessary materials:
Raspberr Pi Pico
Led
10k Potansiyometre
Breadbord
Jumper Kablolar
Circuit diagram
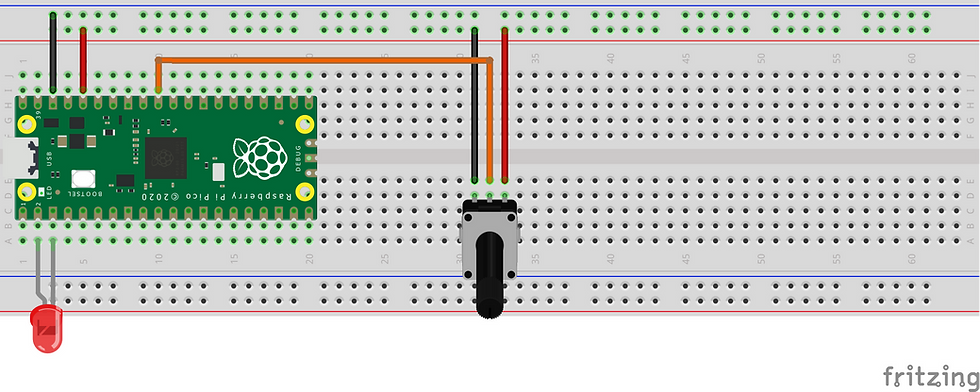
I used a blue led on the circuit and did not use a resistor, because the pico can output 3.3v and the working voltage of the blue led is 3.5v.
CODE
from machine import Pin,PWM,ADC
from time import sleep
led=PWM(Pin(1))
led.freq(1000)
pot=ADC(26)
while True:
pot_value=pot.read_u16()
led.duty_u16(pot_value)
sleep(0.001)
print(pot_value)
sleep(0.01)
led=PWM(Pin(1))
led.freq(1000)
After defining the led pin in this part of the code, we set the output frequency of the pin by typing "led.freq(1000)". The use of this structure is as follows.
pin name.freq(operating frequency)
In our code, the pin name is determined as 1000Hz in the LED operating frequency.
led.duty_u16(pot_value)
Thanks to this code vlog, we can determine the duty rate for the relevant pin. As explained in the "duty_u16" phrase, we can enter a 16-bit value in the parentheses, that is, in the part that says "pot_value". In other words, the PWM pin can take a value between 0 and 65535..
Comments